1.2. Key Computing Ideas
This book is an introduction to computer science, with a focus on the learning of computational concepts and problem-solving skills using the C# programming language.
1.2.1. The Workflow
The workflow that application developers and software engineers use could include the following steps:
Describing a problem scenario to define the goals and objectives.
Plan and organize the features of the computer program that would solve the problem.
Writing the source code of the program using code editors.
Compile the source code into object code as a standalone executable program, usually with the help of a Software Development Kit (SDK) for linking with required resources.
Execute the program and debug to ensure the program runs as intended.
1.2.2. The IPO Model
The input–process–output (IPO) model is widely used in systems analysis and software engineering for describing the structure of an information processing task or process. Computers are used to process mathematical calculations, automate business decisions, and analyze textual data to generate language models, and so on. But in a computer system, these tasks all follow the same structure and go through the same stages. For example, when performing a Google search, you give an input to the search engine, Google processes it, and return back answers to you. That’s what computer systems do: They take in information, process the information, and output the resulted outcome.
1.2.3. Programming Constructs
To learn a natural language, we may start with some basic words for daily usage. Similarly, when it comes to programming languages, we also start with the basic elements of programming such as how computers represents numbers, letters, words, and perform arithmetic operations. Next, we learn the vocabulary of programming, including terms like statement, operator, expression, value, and type [1]. We then put the vocabulary together according to the syntax of a programming language to give instructions to the computers to do the work for us.
A computer program is a set of instructions (written in the specific notations specified by a programming language) given to computers. Interestingly, there are only a small number of ideas that we need to know when learning how to giving instructions to to computers, across probably all programming languages. These basic control structure constructs include:
sequence: instructions are executed one after another (sequential execution).
selection: decision-making/control structure; namely choosing between alternative paths of actions within a program.
iteration: code repetition; either count-controlled or condition-controlled.
Supplementing to the three basic programming constructs are constructs such as:
subroutine: blocks of code in a modular program performing a particular task.
nesting: Selection and iteration constructs can be nested within each other.
variable: named computer memory location that stores values
data type (type): a classification of data values specifying the values and operations on the values.
operator: symbols that perform operations on one or more operands.
array: storing multiple values of the same data type in a single variable.
1.2.4. Problem-solving & Algorithm
Computer science is a scientific discipline, so learning to program could mean learning a new way of thinking; as Allen Downey [1] puts it, to “think like a computer scientist.” As Downey says, “this way of thinking combines some of the best features of mathematics, engineering, and natural science. Like mathematicians, computer scientists use formal languages to denote ideas – specifically computations. Like engineers, they design things, assembling components into systems and evaluating trade-offs among alternatives. Like scientists, they observe the behavior of complex systems, form hypotheses, and test predictions” [1].
For most learners, the idea central in learning computer science and information systems is problem-solving, meaning “the ability to formulate problems, think creatively about solutions, and express a solution clearly and accurately,” [f#2]_ and we expect to hon our problem-solving skills through learning how to code and, in turn, obtaining a skill set to use and create computer programs and tools to solve problems.
In computer science, the solution to a problem is called algorithm, which is a list of step-by-step instructions, much like a recipe for cooking a dish, that will solve the problem under consideration if followed exactly. Programming is the process of implementing an algorithmic solution. By using notations of a programming language, we create a computer program containing specific instructions to the computer to solve the certain problem.
1.2.5. Number Systems
In modern electronic computers are digital systems, meaning they deal with signals (data) that are expressed as series of the digits 0 and 1, the values that represent the state of electrical voltage (on or off). A digit has two states, 0 or 1, and is called a bit. The number system is call binary system, or base 2. A standard data unit is the byte, which is composed of 8 bits, which can represent 256 (2^8, from 0 to 255) different values. In the ASCII (American Standard Code for Information Interchange) code table, the letter “A”, for example, is represented as 0100 0001 in binary number system because it is the 65th symbol in the table. We can translate between the binary and decimal systems as: \((1)2^6 + (0)2^5 + (0)2^4 + (0)2^3 + (0)2^2 + (0)2^1+ (1)2^0 = 64+0+0+0+0+0+1=65\)
Modern computer architecture uses 64-bit long data unit, allowing more data to be processed in CPU and memories. For example, a 32-bit memory address register in teh CPU stores the addresses of the instructions to be fetched from memory. Sine 2^32 is 4,294,967,296, a 32-bit architecture computer therefore has an upper limit of 4 gigabytes for memory. computer to store and work with larger numbers. A 64-bit address register, for example, can address 2^64 different locations, in contrast to a which is why Windows 11 Home supports up to 128GB of RAM while Windows 11 Pro supports up to 2TB of RAM.
1.2.6. Compilation vs. Interpretation
In the early years of computer development, computers only understand low-level languages: machine code (binary digits) to be read and interpreted directly by a computer, and assembly language, consisting of short words to represent machine code instructions. Over time, high-level languages such C, C++, Perl, and Java were created to make programming more efficient. However, the source code written in hight-level programming languages need to be translated into machine code for execution. The two common types of tools for the translation are interpreters and compilers. C#, as a new member of the C-language family , is a compiled programming language. C# source code therefore needs to be compiled to create an executable application to be run by the operating system. Scripting languages such as Bash and Python are interpreted language. They have an interpreter sitting in between the source code and the OS for translation and does not require compilation.
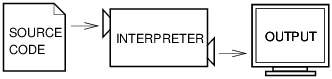
An interpreter processes the program a little at a time, alternately reading lines and performing computations. [3]

A compiler translates source code into object code, which is run by a hardware executor. [3]
Advancement in computing has brought new techniques such as just-in-time (JIT) compilation (dynamic compilation) to combine advantages of traditional interpretation and compilation. Source code is compiled into an intermediate code called bytecode to be interpreted by a virtual machine , then compiled into machine code for faster execution. Many contemporary languages, such as all .NET languages (including C#), Java, Python, and PHP use JIT compilers [4].
1.2.7. Expression & Statements
In programming, an expression is something which evaluates to a value; while a statement is a program instruction. An expression consists of operators and operands as seen in the figure below.
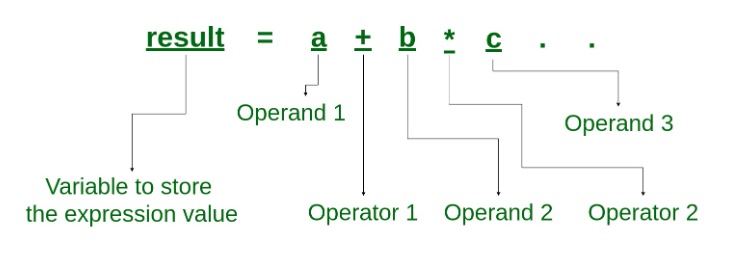
There are different types of expressions in programming. Among them, relational expressions (also called Boolean expressions) and logical expressions are used in selection statements. Relational expressions yield results of type bool which takes a value true or false; while logical expressions combine two or more relational expressions and produces bool type results.
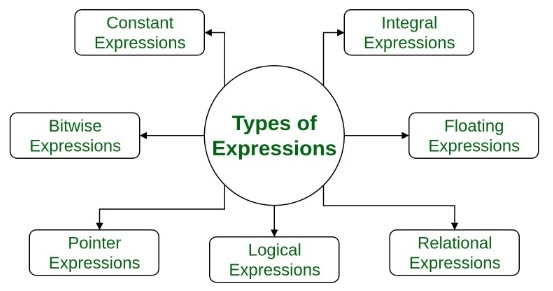
Types of Expressions
1.2.7.1. Statements
A statement performs certain action and usually consists a single line of code that ends in a semicolon, or a series of single-line statements in a block. A statement block is enclosed in {} brackets. Common actions include declaring variables, assigning values, calling methods, looping through collections, and branching to one or another block of code, depending on a given condition.
Some commonly used statement keywords in C# include:
Declaration statements |
|
Selection statements |
if, switch |
Iteration statements |
do, for, foreach, while |
Jump statements |
break, continue, goto, return |
1.2.8. Handling Errors
We will surely make mistakes when learning how to program. The three common types of errors in programming and the ways we handle are:
Syntax Error: These errors happen when the syntax of a programming language is not followed correctly. For compiled languages, syntax errors are detected at compile-time and a program will not compile until all syntax errors are corrected. Syntax errors therefore are often referred to as compile time errors.
Run-Time Error: These errors occur after a program is compiled successfully and while a program is running, often due to memory issues or improper data types. This type of errors are often referred as “bugs”.
Logical Error: Also known as semantic errors, these errors occur when the code is syntactically correct, will not produce a runtime error, but produces incorrect or unexpected results. This could be due to flawed logic, incorrect algorithmic implementation, or other issues.